在项目中一般都会遇到文件的读写,
一般有两个问题要进行处理
1路径问题
2读写问题
路径的解决方法
路径之间的连接”//”=”\”=”/”
eg1:D盘下面file文件夹里面的1.txt
path=”D://file//1.txt”
path=”D:/file/1.txt”
path=”D:\file\1.txt”
这三种都可以
1绝对路径(坚决不推荐使用)
就是从电脑的根目录开始C盘D盘,详情参考eg1
2相对路径
java项目中默认是从项目的根目录开始的 如下图
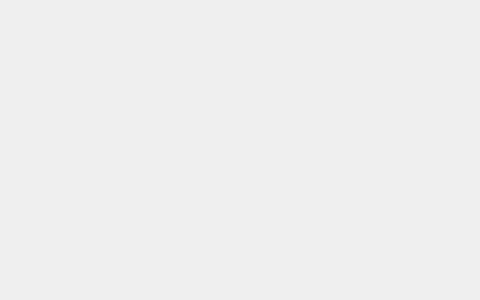
获取到该目录下的所有文件(获取的是一个目录)
./ 获取到当前根目录
- String path=”./”;
- File f=new File(path);
- File[] files=f.listFiles();
- for(int i=0;i<files.length;i++){
- System.out.println(files[i].getName());
- }
../ 获取到根目录下的父目录 想要获取到多级的父目录只需要../ 写n个就好了(需要注意的是这种方法最大只能获取到 Windows盘下面的根目录,就是最多只能获取到 C盘 或者D盘,不可能和Linux 那种 /root/D)web 项目中
主要是分清楚 工作空间和发布空间就好了
比如当初使用struts2文件上传的时候
定义接受文件的目录
ServletContext servletContext = ServletActionContext.getServletContext();
String str=servletContext.getRealPath(“/files/”+fileFileName);
eclipse暂时出了点小问题等会再写这个
读写文件(如果不正确欢迎积极指出,一起进步)
因为文件有不同的格式,就文本文件来说有utf-8 GBK 等等
建议使用字节流 ( InputStream是所有字节输入流的祖先,而OutputStream是所有字节输出流的祖先)进行读取而不是字符流( Reader是所有读取字符串输入流的祖先,而writer是所有输出字符串的祖先)
其实就是内部一个使用byte[]实现,一个是用char[] 这个可以看一下 JDK的源码就了解了
具体 字符流字节流之间的区别请看转载处
- http://blog.csdn.net/zxman660/article/details/7875799
- http://blog.csdn.net/cynhafa/article/details/6882061
- 读写文件
- package com.wzh.utils;
- import java.io.BufferedInputStream;
- import java.io.BufferedOutputStream;
- import java.io.File;
- import java.io.FileInputStream;
- import java.io.FileOutputStream;
- import java.io.InputStream;
- import java.io.OutputStream;
- public class InOutFile {
- /**
- * @param file null、一个文件、一个文件目录、
- * <pre>
- * fileToByte(null)=null
- * fileToByte(file)=null file>2G
- * fileToByte(文件目录)=null
- * fileToByte(file)=byte[]
- * </pre>
- * @return null(文件不存在,null,文件目录,文件太大>2G) byte[](转换成功)
- */
- public byte[] fileToByte(File file) {
- byte[] buffer = null;
- if (file == null) {
- throw new IndexOutOfBoundsException();
- }
- if (file.isDirectory())
- return buffer;
- if (file.length() > Integer.MAX_VALUE)
- return buffer;
- if (file.isFile()) {
- int filelength = (int) file.length();
- InputStream inputStream = null;
- BufferedInputStream bufferedInputStream = null;
- OutputStream outputStream=null;
- BufferedOutputStream bufferedOutputStream=null;
- File outfile=new File(“files//out//”+file.getName());
- int n = 0;
- int off = 0;
- int length = 4096;
- try {
- if(!outfile.exists())
- outfile.createNewFile();
- inputStream = new FileInputStream(file);
- outputStream=new FileOutputStream(outfile, true);
- bufferedInputStream = new BufferedInputStream(inputStream);
- bufferedOutputStream=new BufferedOutputStream(outputStream);
- buffer = new byte[filelength];
- /*
- * 添加(length <= filelength – off) ? length : filelength – off)的比较
- * 为了防止读到最后buffer 剩余的长度没有4096 装不下那么多会导致读取不了IndexOutOfBoundsException()
- * 当filelength – off=0时表示文件读取完毕但是read内部认为是其他线程占用io导致堵塞并不会认为文件读取完毕
- * 所以要添加上filelength – off>0
- */
- while ((filelength – off) > 0 && (n = bufferedInputStream.read(buffer, off,
- ((length <= filelength – off) ? length : filelength – off))) >= 0) {
- bufferedOutputStream.write(buffer, off, n);
- off += n;
- }
- }
- catch (Exception e) {
- }
- finally {
- closeInputStream(bufferedInputStream);
- closeInputStream(inputStream);
- closeOutputStream(bufferedOutputStream);
- closeOutputStream(outputStream);
- System.out.println(“end”);
- }
- }
- return buffer;
- }
- /**
- * close inoutstream
- * @param inputStream null or the inputstream’s child
- */
- private void closeInputStream(InputStream inputStream) {
- if (inputStream == null)
- return;
- try {
- inputStream.close();
- }
- catch (Exception e) {
- }
- }
- /**
- * close outputStream
- * @param outputStream null or the outputStream child
- */
- private void closeOutputStream(OutputStream outputStream) {
- if (outputStream == null)
- return;
- try {
- outputStream.flush();
- outputStream.close();
- }
- catch (Exception e) {
- }
- }
- }
当然字符流也可以进行读取文件
字符流也可以进行读取文件只不过要指定文件(文本文件)的编码
- /**
- * 创建不同格式的文本文件
- * @throws Exception
- */
- private void createFile() throws Exception {
- File file = new File(“files//kindsformat//utf//1.txt”);
- //File file = new File(“files//kindsformat//gbk//1.txt”);
- if (!file.exists())
- file.createNewFile();
- Writer writer = new OutputStreamWriter(new FileOutputStream(file, true), “UTF-8”);
- //Writer writer = new OutputStreamWriter(new FileOutputStream(file), “GBK”);
- BufferedWriter bufferedWriter = new BufferedWriter(writer);
- bufferedWriter.write(“我是中文测试啊测试啊”);
- bufferedWriter.flush();
- bufferedWriter.close();
- writer.close();
- }
有不对的地方欢迎指出,谢谢
版权声明:本文内容由互联网用户自发贡献,该文观点仅代表作者本人。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如发现本站有涉嫌抄袭侵权/违法违规的内容, 请发送邮件至 举报,一经查实,本站将立刻删除。